Atomic cluster expansion (ACE)
Contents
Atomic cluster expansion (ACE)#
Please read sources for more details:
Import and load#
%pylab inline
%pylab is deprecated, use %matplotlib inline and import the required libraries.
Populating the interactive namespace from numpy and matplotlib
from pyiron import Project
from collections import Counter
import pandas as pd
pr = Project('fit_pace')
#pr.remove_jobs(silently=True)
pr_data = Project("../../introduction/training")
Loading training containers (select smaller training subset for example)#
training_container = pr_data['full']
df=training_container.to_pandas()
df["energy_per_atom"]=df["energy"]/df["number_of_atoms"]
df["comp_dict"]=df["atoms"].map(lambda at: Counter(at.get_chemical_symbols()))
for el in ["Al","Li"]:
df["n"+el]=df["comp_dict"].map(lambda d: d.get(el,0))
df["c"+el]=df["n"+el]/df["number_of_atoms"]
Select only Al-fcc
al_fcc_df = df[df["name"].str.contains("Al_fcc")]
al_fcc_df.shape
(134, 12)
only Li-bcc
li_bcc_df = df[df["name"].str.contains("Li_bcc")]
li_bcc_df.shape
(179, 12)
and AlLi structures that are within 0.1 eV/atom above AlLi ground state (min.energy structure)
alli_df = df[df["cAl"]==0.5]
alli_df=alli_df[alli_df["energy_per_atom"]<=alli_df["energy_per_atom"].min()+0.1]
alli_df.shape
(178, 12)
small_training_df = pd.concat([al_fcc_df, li_bcc_df, alli_df])
small_training_df.shape
(491, 12)
small_training_df["number_of_atoms"].hist(bins=100);
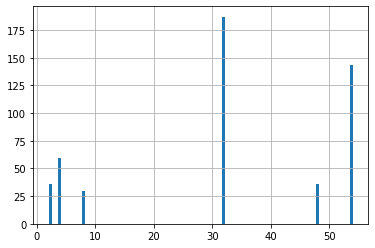
Select only sctructures smaller than 40 atoms per structures
small_training_df=small_training_df[small_training_df["number_of_atoms"]<=40].sample(n=100, random_state=42)
small_training_df.shape
(100, 12)
Pack them into training container
small_tc = pr.create.job.TrainingContainer("small_AlLi_training_container", delete_existing_job=True)
small_tc.include_dataset(small_training_df)
small_tc.save()
The job small_AlLi_training_container was saved and received the ID: 27
27
small_tc.plot.energy_volume(crystal_systems=True)
V | E | space_group | crystal_system | |
---|---|---|---|---|
0 | 18.537039 | -2.714437 | 194 | hexagonal |
1 | 17.014115 | -3.415845 | 1 | triclinic |
2 | 18.132856 | -3.449419 | 225 | cubic |
3 | 18.512802 | -3.413774 | 1 | triclinic |
4 | 20.298508 | -3.178243 | 1 | triclinic |
... | ... | ... | ... | ... |
95 | 15.707664 | -2.800103 | 227 | cubic |
96 | 18.722061 | -3.399934 | 1 | triclinic |
97 | 16.388387 | -2.746841 | 1 | triclinic |
98 | 16.697821 | -3.450689 | 1 | triclinic |
99 | 16.484904 | -3.482749 | 225 | cubic |
100 rows × 4 columns
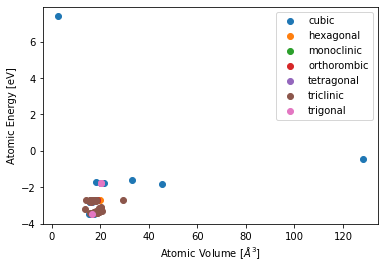
Create PacemakerJob#
job = pr.create.job.PacemakerJob("pacemaker_job")
job.add_training_data(small_tc)
Set maximum cutoff for the potential
PACE potential fitting setup#
Overview of settings#
job.input
Parameter | Value | Comment | |
---|---|---|---|
0 | cutoff | 7.0 | |
1 | metadata | {'comment': 'pyiron-generated fitting job'} | |
2 | data | {} | |
3 | potential | {'elements': [], 'bonds': {'ALL': {'radbase': 'SBessel', 'rcut': 7.0, 'dcut': 0.01, 'radparameters': [5.25]}}, 'embeddings': {'ALL': {'fs_parameters': [1, 1, 1, 0.5], 'ndensity': 2, 'npot': 'Finni... | |
4 | fit | {'loss': {'L1_coeffs': 1e-08, 'L2_coeffs': 1e-08, 'kappa': 0.3, 'w0_rad': 0, 'w1_rad': 0, 'w2_rad': 0}, 'maxiter': 1000, 'optimizer': 'BFGS', 'fit_cycles': 1} | |
5 | backend | {'batch_size': 100, 'display_step': 50, 'evaluator': 'tensorpot'} |
Potential interatomic distance cutoff#
job.cutoff=7.0
Specification of ACE potential#
PACE potential specification consists of three parts:
1. Embeddings#
i.e. how atomic energy \(E_i\) depends on ACE properties/densities \(\varphi\). Linear expansion \(E_i = \varphi\) is the trivial. Non-linear expansion, i.e. those, containing square root, gives more flexiblity and accuracy of final potential
Embeddings for ALL
species (i.e. Al and Li):
non-linear
FinnisSinclairShiftedScaled
2 densities
fs_parameters’: [1, 1, 1, 0.5]: $\(E_i = 1.0 * \varphi(1)^1 + 1.0 * \varphi(2)^{0.5} = \varphi^{(1)} + \sqrt{\varphi^{(2)}} \)$
job.input["potential"]["embeddings"]
{'ALL': {'fs_parameters': [1, 1, 1, 0.5],
'ndensity': 2,
'npot': 'FinnisSinclairShiftedScaled'}}
2. Radial functions#
Radial functions are orthogonal polynoms example:
(a) Exponentially-scaled Chebyshev polynomials (λ = 5.25)
(b) Power-law scaled Chebyshev polynomials (λ = 2.0)
(c) Simplified spherical Bessel functions
Radial functions specification for ALL
species pairs (i.e. Al-Al, Al-Li, Li-Al, Li-Li):
based on the Simplified Bessel
cutoff \(r_c=7.0\)
job.input["potential"]["bonds"]
{'ALL': {'radbase': 'SBessel',
'rcut': 7.0,
'dcut': 0.01,
'radparameters': [5.25]}}
3. B-basis functions#
B-basis functions for ALL
species type interactions, i.e. Al-Al, Al-Li, Li-Al, Li-Li blocks:
maximum order = 4, i.e. body-order 5 (1 central atom + 4 neighbour densities)
nradmax_by_orders: 15, 3, 2, 1
lmax_by_orders: 0, 3, 2, 1
job.input["potential"]["functions"]
{'ALL': {'nradmax_by_orders': [15, 3, 2, 1], 'lmax_by_orders': [0, 3, 2, 1]}}
We will reduce the basis size for demonstartion purposes
job.input["potential"]["functions"]={'ALL': {'nradmax_by_orders': [15, 3, 2], 'lmax_by_orders': [0, 2, 1]}}
Fit/loss specification#
Loss function specification
job.input["fit"]['loss']
{'L1_coeffs': 1e-08,
'L2_coeffs': 1e-08,
'kappa': 0.3,
'w0_rad': 0,
'w1_rad': 0,
'w2_rad': 0}
Weighting#
Energy-based weighting puts more “accent” onto the low energy-lying structures, close to convex hull
job.input["fit"]['weighting'] = {
## weights for the structures energies/forces are associated according to the distance to E_min:
## convex hull ( energy: convex_hull) or minimal energy per atom (energy: cohesive)
"type": "EnergyBasedWeightingPolicy",
## number of structures to randomly select from the initial dataset
"nfit": 10000,
## only the structures with energy up to E_min + DEup will be selected
"DEup": 10.0, ## eV, upper energy range (E_min + DElow, E_min + DEup)
## only the structures with maximal force on atom up to DFup will be selected
"DFup": 50.0, ## eV/A
## lower energy range (E_min, E_min + DElow)
"DElow": 1.0, ## eV
## delta_E shift for weights, see paper
"DE": 1.0,
## delta_F shift for weights, see paper
"DF": 1.0,
## 0<wlow<1 or None: if provided, the renormalization weights of the structures on lower energy range (see DElow)
"wlow": 0.75,
## "convex_hull" or "cohesive" : method to compute the E_min
"energy": "convex_hull",
## structures types: all (default), bulk or cluster
"reftype": "all",
## random number seed
"seed": 42
}
Minimization and backend specification#
Type of optimizer: SciPy.minimize.BFGS
. This optimizer is more efficient that typical optimizers for neural networks (i.e. ADAM, RMSprop, Adagrad, etc.), but it scales quadratically wrt. number of optimizable parameters.
However number of trainable parameters for PACE potential is usually up to few thousands, so we gain a lot of accuracy during training with BFGS optimizer.
job.input["fit"]["optimizer"]
'BFGS'
Maximum number of iterations by minimizer. Typical values are ~1000-1500, but we chose small value for demonstration purposes only
job.input["fit"]["maxiter"]=100
Batch size (number of simultaneously considered structures). This number should be reduced if there is not enough memory
job.input["backend"]["batch_size"]
100
For more details about these and other settings please refer to official documentation
Run fit#
job.run()
INFO:pyiron_log:run job: pacemaker_job id: 28, status: created
INFO:pyiron_log:job: pacemaker_job id: 28, status: submitted, run job (modal)
Set automatically determined list of elements: ['Al', 'Li']
The job pacemaker_job was saved and received the ID: 28
INFO:pyiron_log:job: pacemaker_job id: 28, status: collect, output: /srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:499: UserWarning: The value of the smallest subnormal for <class 'numpy.float64'> type is zero.
setattr(self, word, getattr(machar, word).flat[0])
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:89: UserWarning: The value of the smallest subnormal for <class 'numpy.float64'> type is zero.
return self._float_to_str(self.smallest_subnormal)
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:499: UserWarning: The value of the smallest subnormal for <class 'numpy.float32'> type is zero.
setattr(self, word, getattr(machar, word).flat[0])
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:89: UserWarning: The value of the smallest subnormal for <class 'numpy.float32'> type is zero.
return self._float_to_str(self.smallest_subnormal)
2022-06-06 19:44:42,455 - root - INFO - Redirecting log into file log.txt
2022-06-06 19:44:42,455 - root - INFO - Start pacemaker
2022-06-06 19:44:42,455 - root - INFO - pacemaker/pyace version: 0.2.7+49.gac4f57f
2022-06-06 19:44:42,455 - root - INFO - ace_evaluator version: 2021.10.25
2022-06-06 19:44:42,455 - root - INFO - Loading input.yaml...
2022-06-06 19:44:42,462 - root - WARNING - No 'seed' provided in YAML file, default value seed = 42 will be used.
2022-06-06 19:44:42,462 - pyace.generalfit - INFO - Set numpy random seed to 42
2022-06-06 19:44:42,632 - pyace.generalfit - INFO - Target potential shape constructed from dictionary, it contains 306 functions
2022-06-06 19:44:43,834 - pyace.generalfit - INFO - tensorpot_version: 0+untagged.8.g783e6a6
2022-06-06 19:44:43,834 - pyace.generalfit - INFO - User name automatically identified: jovyan
2022-06-06 19:44:43,834 - pyace.preparedata - INFO - Search for cache ref-file: /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/df_fit.pckl.gzip
2022-06-06 19:44:43,834 - pyace.preparedata - INFO - /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/df_fit.pckl.gzip found, try to load
2022-06-06 19:44:43,834 - pyace.preparedata - INFO - Loading dataframe from pickle file /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/df_fit.pckl.gzip (101.4KiB)
2022-06-06 19:44:43,838 - pyace.preparedata - INFO - Transforming to DataFrameWithMetadata
2022-06-06 19:44:43,838 - pyace.preparedata - INFO - Setting up structures dataframe - please be patient...
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - Processing structures dataframe. Shape: (100, 6)
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - Total number of atoms: 2240
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - Mean number of atoms per structure: 22.4
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - 'energy' columns found
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - 'forces' columns found
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - 'pbc' columns found
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - 'cell' column found
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - 'energy_corrected' column found
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - 'energy_corrected_per_atom' column extraction
2022-06-06 19:44:43,839 - pyace.preparedata - INFO - Min energy per atom: -3.483 eV/atom
2022-06-06 19:44:43,840 - pyace.preparedata - INFO - Max energy per atom: 7.379 eV/atom
2022-06-06 19:44:43,840 - pyace.preparedata - INFO - Min abs energy per atom: 0.444 eV/atom
2022-06-06 19:44:43,840 - pyace.preparedata - INFO - Max abs energy per atom: 7.379 eV/atom
2022-06-06 19:44:43,840 - pyace.preparedata - INFO - ASE atoms ('ase_atoms' column) are already in dataframe
2022-06-06 19:44:43,840 - pyace.preparedata - INFO - Attaching SinglePointCalculator to ASE atoms...
2022-06-06 19:44:43,847 - pyace.preparedata - INFO - Attaching SinglePointCalcualtor to ASE atoms...done within 0.00669026 sec (0.00298672 ms/at)
2022-06-06 19:44:43,847 - pyace.preparedata - INFO - Atomic environment representation construction...
2022-06-06 19:44:43,847 - pyace.preparedata - INFO - Building 'tp_atoms' (dataset size 100, cutoff=7.0A)...
2022-06-06 19:44:43,939 - pyace.preparedata - INFO - Dataframe size after transform: 100
2022-06-06 19:44:43,939 - pyace.preparedata - INFO - Atomic environment representation construction...done within 0.092207 sec (0.0412 ms/atom)
2022-06-06 19:44:43,939 - pyace.preparedata - INFO - Saving processed raw dataframe into /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/df_fit.pckl.gzip
2022-06-06 19:44:43,939 - pyace.preparedata - INFO - Writing fit pickle file: /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/df_fit.pckl.gzip
2022-06-06 19:44:44,886 - pyace.preparedata - INFO - Saved to file /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/df_fit.pckl.gzip (486.3KiB)
2022-06-06 19:44:44,886 - pyace.preparedata - INFO - Apply weights policy: EnergyBasedWeightingPolicy(nfit=10000, energy=convex_hull, DElow=1.0, DEup=10.0, DFup=50.0, DE=1.0, DF=1.0, wlow=0.75, reftype=all,seed=42)
2022-06-06 19:44:44,886 - pyace.preparedata - INFO - Keeping bulk and cluster data
2022-06-06 19:44:44,886 - pyace.preparedata - INFO - No cutoff for EnergyBasedWeightingPolicy is provided, no structures outside cutoff that will now be removed
2022-06-06 19:44:44,886 - pyace.preparedata - INFO - EnergyBasedWeightingPolicy: energy reference frame - convex hull distance (if possible)
2022-06-06 19:44:44,891 - pyace.preparedata - INFO - Pure element lowest energy for Al = -3.482749 eV/atom
2022-06-06 19:44:44,891 - pyace.preparedata - INFO - Pure element lowest energy for Li = -1.756339 eV/atom
2022-06-06 19:44:44,892 - pyace.preparedata - INFO - Structure dataset: multiple unique compositions found, trying to construct convex hull
2022-06-06 19:44:44,894 - pyace.preparedata - INFO - Maximal allowed on-atom force vector length is DFup = 50.000
2022-06-06 19:44:44,896 - pyace.preparedata - INFO - 0 structures with higher than dFup forces are removed. Current size: 100 structures
2022-06-06 19:44:44,896 - pyace.preparedata - INFO - 97 structures below DElow=1.0 eV/atom
2022-06-06 19:44:44,896 - pyace.preparedata - INFO - 3 structures between DElow=1.0 eV/atom and DEup=10.0 eV/atom
2022-06-06 19:44:44,896 - pyace.preparedata - INFO - all other structures were removed
2022-06-06 19:44:44,897 - pyace.preparedata - INFO - 100 structures were selected
2022-06-06 19:44:44,897 - pyace.preparedata - INFO - Setting up energy weights
2022-06-06 19:44:44,898 - pyace.preparedata - INFO - Current relative energy weights: 0.996/0.004
2022-06-06 19:44:44,898 - pyace.preparedata - INFO - Will be adjusted to : 0.750/0.250
2022-06-06 19:44:44,899 - pyace.preparedata - INFO - After adjustment: relative energy weights: 0.750/0.250
2022-06-06 19:44:44,899 - pyace.preparedata - INFO - Setting up force weights
2022-06-06 19:44:44,927 - pyace.generalfit - INFO - Fitting dataset info saved into fitting_data_info.pckl.gzip
2022-06-06 19:44:44,927 - pyace.generalfit - INFO - Plotting train energy-forces distribution
2022-06-06 19:44:45,371 - pyace.generalfit - INFO - Fitting dataset size: 100 structures / 2240 atoms
2022-06-06 19:44:45,372 - pyace.generalfit - INFO - 'Single-shot' fitting
2022-06-06 19:44:45,372 - pyace.generalfit - INFO - Cycle fitting loop
2022-06-06 19:44:45,372 - pyace.generalfit - INFO - Number of fit attempts: 0/1
2022-06-06 19:44:45,372 - pyace.generalfit - INFO - Total number of functions: 306 / number of parameters: 1152
2022-06-06 19:44:45,372 - pyace.generalfit - INFO - Running fit backend
2022-06-06 19:44:45,509 - pyace.fitadapter - INFO - Trainable parameters: {('Al',): ['func', 'radial'], ('Li',): ['func', 'radial'], ('Al', 'Li'): ['func', 'radial'], ('Li', 'Al'): ['func', 'radial']}
2022-06-06 19:44:45,510 - pyace.fitadapter - INFO - Loss function specification: LossFunctionSpecification(kappa=0.3, L1=1e-08, L2=1e-08, DeltaRad=(0, 0, 0), w_orth=0)
2022-06-06 19:44:45,510 - pyace.fitadapter - INFO - Batch size: 100
2022-06-06 19:44:45,828 - tensorpotential.fit - INFO - Minimizer options: {'gtol': 1e-08, 'disp': True, 'maxiter': 100}
2022-06-06 19:44:50,331 - tensorflow - WARNING - Calling GradientTape.gradient on a persistent tape inside its context is significantly less efficient than calling it outside the context (it causes the gradient ops to be recorded on the tape, leading to increased CPU and memory usage). Only call GradientTape.gradient inside the context if you actually want to trace the gradient in order to compute higher order derivatives.
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("concat_55:0", shape=(None,), dtype=int32), values=Tensor("concat_54:0", shape=(None, 3), dtype=float64), dense_shape=Tensor("gradient_tape/Cast_30:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_493:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_492:0", shape=(None, 15), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_4:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_495:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_494:0", shape=(None, 15), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_5:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_497:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_496:0", shape=(None, 15), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_6:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_499:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_498:0", shape=(None, 15), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_7:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_538:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_537:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_9:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_540:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_539:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_10:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_542:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_541:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_11:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_544:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_543:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_12:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_546:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_545:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_13:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_548:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_547:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_14:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_550:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_549:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_15:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_552:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_551:0", shape=(None, 3, 9), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_16:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_636:0", shape=(126,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_635:0", shape=(126, None), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_17:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_638:0", shape=(126,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_637:0", shape=(126, None), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_18:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_644:0", shape=(100,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_643:0", shape=(100, None), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_19:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/gradient_tape/Reshape_646:0", shape=(100,), dtype=int32), values=Tensor("gradient_tape/gradient_tape/Reshape_645:0", shape=(100, None), dtype=float64), dense_shape=Tensor("gradient_tape/gradient_tape/Cast_20:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
2022-06-06 19:45:35,157 - pyace.metrics_aggregator - INFO - Initial state:#0 (1 evals): Loss: 5.271737 | RMSE Energy(low): 3037.77 (2985.74) meV/at | Forces(low): 376.89 (377.56) meV/A | Time/eval: 22021.47 mcs/at
2022-06-06 19:45:35,158 - pyace.metrics_aggregator - INFO -
--------------------------------------------INIT STATS--------------------------------------------
Iteration: #0 Loss: Total: 5.2717e+00 (100%)
Energy: 5.2034e+00 ( 99%)
Force: 6.8301e-02 ( 1%)
L1: 0.0000e+00 ( 0%)
L2: 0.0000e+00 ( 0%)
Number of params./funcs: 1017/306 Avg. time: 22021.47 mcs/at
-------------------------------------------------------------------------------------------------
Energy/at, meV/at Energy_low/at, meV/at Force, meV/A Force_low, meV/A
RMSE: 3037.77 2985.74 376.89 377.56
MAE: 2954.99 2946.61 248.06 248.95
MAX_AE: 7378.77 3482.75 2865.76 2865.76
-------------------------------------------------------------------------------------------------
2022-06-06 19:45:38,756 - pyace.metrics_aggregator - INFO - Iteration #1 (4 evals): Loss: 5.006615 | RMSE Energy(low): 2948.73 (2986.03) meV/at | Forces(low): 376.84 (377.51) meV/A | Time/eval: 533.62 mcs/at
2022-06-06 19:45:41,346 - pyace.metrics_aggregator - INFO - Iteration #2 (6 evals): Loss: 4.942739 | RMSE Energy(low): 2929.51 (2958.17) meV/at | Forces(low): 376.23 (376.90) meV/A | Time/eval: 490.94 mcs/at
2022-06-06 19:45:43,953 - pyace.metrics_aggregator - INFO - Iteration #3 (8 evals): Loss: 4.357858 | RMSE Energy(low): 2740.29 (2769.61) meV/at | Forces(low): 372.26 (372.93) meV/A | Time/eval: 533.27 mcs/at
2022-06-06 19:45:45,361 - pyace.metrics_aggregator - INFO - Iteration #4 (9 evals): Loss: 3.329202 | RMSE Energy(low): 2375.69 (2396.63) meV/at | Forces(low): 362.07 (362.72) meV/A | Time/eval: 531.90 mcs/at
2022-06-06 19:45:47,848 - pyace.metrics_aggregator - INFO - Iteration #5 (11 evals): Loss: 2.484392 | RMSE Energy(low): 2049.08 (2029.83) meV/at | Forces(low): 326.13 (326.71) meV/A | Time/eval: 531.97 mcs/at
2022-06-06 19:45:49,160 - pyace.metrics_aggregator - INFO - Iteration #6 (12 evals): Loss: 1.437047 | RMSE Energy(low): 1538.12 (1522.19) meV/at | Forces(low): 271.95 (272.44) meV/A | Time/eval: 490.89 mcs/at
2022-06-06 19:45:51,655 - pyace.metrics_aggregator - INFO - Iteration #7 (14 evals): Loss: 0.650425 | RMSE Energy(low): 915.39 (900.98) meV/at | Forces(low): 376.73 (377.41) meV/A | Time/eval: 491.53 mcs/at
2022-06-06 19:45:55,162 - pyace.metrics_aggregator - INFO - Iteration #8 (17 evals): Loss: 0.569036 | RMSE Energy(low): 823.89 (771.34) meV/at | Forces(low): 389.28 (389.98) meV/A | Time/eval: 451.54 mcs/at
2022-06-06 19:45:56,558 - pyace.metrics_aggregator - INFO - Iteration #9 (18 evals): Loss: 0.429327 | RMSE Energy(low): 714.60 (668.29) meV/at | Forces(low): 299.24 (299.78) meV/A | Time/eval: 493.90 mcs/at
2022-06-06 19:45:57,857 - pyace.metrics_aggregator - INFO - Iteration #10 (19 evals): Loss: 0.242773 | RMSE Energy(low): 442.61 (427.93) meV/at | Forces(low): 364.51 (365.17) meV/A | Time/eval: 480.00 mcs/at
2022-06-06 19:46:00,257 - pyace.metrics_aggregator - INFO - Iteration #11 (21 evals): Loss: 0.211923 | RMSE Energy(low): 424.97 (421.42) meV/at | Forces(low): 345.77 (346.39) meV/A | Time/eval: 490.33 mcs/at
2022-06-06 19:46:01,556 - pyace.metrics_aggregator - INFO - Iteration #12 (22 evals): Loss: 0.180375 | RMSE Energy(low): 389.46 (373.98) meV/at | Forces(low): 345.03 (345.65) meV/A | Time/eval: 463.67 mcs/at
2022-06-06 19:46:02,855 - pyace.metrics_aggregator - INFO - Iteration #13 (23 evals): Loss: 0.160687 | RMSE Energy(low): 342.90 (342.94) meV/at | Forces(low): 368.44 (369.10) meV/A | Time/eval: 471.37 mcs/at
2022-06-06 19:46:04,157 - pyace.metrics_aggregator - INFO - Iteration #14 (24 evals): Loss: 0.137387 | RMSE Energy(low): 328.82 (307.94) meV/at | Forces(low): 349.99 (350.62) meV/A | Time/eval: 484.52 mcs/at
2022-06-06 19:46:05,553 - pyace.metrics_aggregator - INFO - Iteration #15 (25 evals): Loss: 0.101963 | RMSE Energy(low): 274.62 (266.59) meV/at | Forces(low): 318.81 (319.39) meV/A | Time/eval: 511.49 mcs/at
2022-06-06 19:46:07,956 - pyace.metrics_aggregator - INFO - Iteration #16 (27 evals): Loss: 0.089982 | RMSE Energy(low): 269.83 (270.00) meV/at | Forces(low): 292.43 (292.95) meV/A | Time/eval: 490.94 mcs/at
2022-06-06 19:46:09,256 - pyace.metrics_aggregator - INFO - Iteration #17 (28 evals): Loss: 0.071076 | RMSE Energy(low): 242.74 (244.92) meV/at | Forces(low): 260.72 (261.19) meV/A | Time/eval: 472.18 mcs/at
2022-06-06 19:46:11,756 - pyace.metrics_aggregator - INFO - Iteration #18 (30 evals): Loss: 0.061088 | RMSE Energy(low): 245.90 (247.09) meV/at | Forces(low): 230.43 (230.84) meV/A | Time/eval: 534.58 mcs/at
2022-06-06 19:46:13,058 - pyace.metrics_aggregator - INFO - Iteration #19 (31 evals): Loss: 0.049180 | RMSE Energy(low): 206.64 (208.90) meV/at | Forces(low): 222.59 (222.99) meV/A | Time/eval: 482.22 mcs/at
2022-06-06 19:46:14,453 - pyace.metrics_aggregator - INFO - Iteration #20 (32 evals): Loss: 0.040131 | RMSE Energy(low): 177.91 (180.40) meV/at | Forces(low): 206.61 (206.98) meV/A | Time/eval: 502.92 mcs/at
2022-06-06 19:46:15,852 - pyace.metrics_aggregator - INFO - Iteration #21 (33 evals): Loss: 0.035315 | RMSE Energy(low): 179.86 (176.07) meV/at | Forces(low): 185.96 (186.29) meV/A | Time/eval: 529.35 mcs/at
2022-06-06 19:46:17,257 - pyace.metrics_aggregator - INFO - Iteration #22 (34 evals): Loss: 0.029719 | RMSE Energy(low): 153.08 (153.33) meV/at | Forces(low): 178.95 (179.27) meV/A | Time/eval: 531.07 mcs/at
2022-06-06 19:46:18,656 - pyace.metrics_aggregator - INFO - Iteration #23 (35 evals): Loss: 0.026540 | RMSE Energy(low): 146.72 (141.06) meV/at | Forces(low): 161.62 (161.91) meV/A | Time/eval: 500.02 mcs/at
2022-06-06 19:46:20,054 - pyace.metrics_aggregator - INFO - Iteration #24 (36 evals): Loss: 0.023045 | RMSE Energy(low): 122.78 (121.77) meV/at | Forces(low): 157.82 (158.10) meV/A | Time/eval: 502.23 mcs/at
2022-06-06 19:46:21,359 - pyace.metrics_aggregator - INFO - Iteration #25 (37 evals): Loss: 0.020847 | RMSE Energy(low): 113.61 (114.20) meV/at | Forces(low): 153.73 (154.01) meV/A | Time/eval: 485.26 mcs/at
2022-06-06 19:46:22,754 - pyace.metrics_aggregator - INFO - Iteration #26 (38 evals): Loss: 0.018143 | RMSE Energy(low): 108.41 (108.71) meV/at | Forces(low): 148.23 (148.49) meV/A | Time/eval: 504.73 mcs/at
2022-06-06 19:46:24,059 - pyace.metrics_aggregator - INFO - Iteration #27 (39 evals): Loss: 0.017013 | RMSE Energy(low): 103.84 (104.84) meV/at | Forces(low): 146.27 (146.53) meV/A | Time/eval: 486.57 mcs/at
2022-06-06 19:46:25,461 - pyace.metrics_aggregator - INFO - Iteration #28 (40 evals): Loss: 0.015253 | RMSE Energy(low): 102.93 (104.12) meV/at | Forces(low): 139.32 (139.57) meV/A | Time/eval: 498.24 mcs/at
2022-06-06 19:46:26,951 - pyace.metrics_aggregator - INFO - Iteration #29 (41 evals): Loss: 0.013546 | RMSE Energy(low): 103.02 (104.52) meV/at | Forces(low): 130.92 (131.15) meV/A | Time/eval: 536.32 mcs/at
2022-06-06 19:46:28,257 - pyace.metrics_aggregator - INFO - Iteration #30 (42 evals): Loss: 0.011605 | RMSE Energy(low): 98.35 (98.75) meV/at | Forces(low): 121.66 (121.88) meV/A | Time/eval: 487.02 mcs/at
2022-06-06 19:46:29,659 - pyace.metrics_aggregator - INFO - Iteration #31 (43 evals): Loss: 0.010090 | RMSE Energy(low): 95.18 (96.58) meV/at | Forces(low): 117.07 (117.28) meV/A | Time/eval: 521.90 mcs/at
2022-06-06 19:46:32,162 - pyace.metrics_aggregator - INFO - Iteration #32 (45 evals): Loss: 0.009337 | RMSE Energy(low): 86.79 (87.21) meV/at | Forces(low): 114.48 (114.69) meV/A | Time/eval: 493.27 mcs/at
2022-06-06 19:46:33,556 - pyace.metrics_aggregator - INFO - Iteration #33 (46 evals): Loss: 0.008232 | RMSE Energy(low): 81.67 (82.53) meV/at | Forces(low): 109.65 (109.85) meV/A | Time/eval: 500.22 mcs/at
2022-06-06 19:46:34,955 - pyace.metrics_aggregator - INFO - Iteration #34 (47 evals): Loss: 0.007628 | RMSE Energy(low): 74.82 (75.71) meV/at | Forces(low): 104.90 (105.08) meV/A | Time/eval: 523.92 mcs/at
2022-06-06 19:46:36,355 - pyace.metrics_aggregator - INFO - Iteration #35 (48 evals): Loss: 0.006763 | RMSE Energy(low): 71.14 (71.96) meV/at | Forces(low): 99.19 (99.37) meV/A | Time/eval: 524.20 mcs/at
2022-06-06 19:46:37,753 - pyace.metrics_aggregator - INFO - Iteration #36 (49 evals): Loss: 0.006352 | RMSE Energy(low): 74.35 (68.15) meV/at | Forces(low): 91.94 (92.10) meV/A | Time/eval: 525.09 mcs/at
2022-06-06 19:46:39,056 - pyace.metrics_aggregator - INFO - Iteration #37 (50 evals): Loss: 0.005737 | RMSE Energy(low): 69.04 (66.37) meV/at | Forces(low): 89.65 (89.81) meV/A | Time/eval: 480.96 mcs/at
2022-06-06 19:46:40,457 - pyace.metrics_aggregator - INFO - Iteration #38 (51 evals): Loss: 0.005069 | RMSE Energy(low): 64.56 (64.15) meV/at | Forces(low): 86.20 (86.35) meV/A | Time/eval: 500.19 mcs/at
2022-06-06 19:46:41,858 - pyace.metrics_aggregator - INFO - Iteration #39 (52 evals): Loss: 0.004368 | RMSE Energy(low): 61.88 (60.27) meV/at | Forces(low): 82.42 (82.56) meV/A | Time/eval: 524.45 mcs/at
2022-06-06 19:46:43,255 - pyace.metrics_aggregator - INFO - Iteration #40 (53 evals): Loss: 0.004100 | RMSE Energy(low): 56.15 (56.34) meV/at | Forces(low): 82.33 (82.48) meV/A | Time/eval: 513.92 mcs/at
2022-06-06 19:46:44,557 - pyace.metrics_aggregator - INFO - Iteration #41 (54 evals): Loss: 0.003814 | RMSE Energy(low): 57.11 (56.58) meV/at | Forces(low): 78.42 (78.56) meV/A | Time/eval: 479.23 mcs/at
2022-06-06 19:46:45,856 - pyace.metrics_aggregator - INFO - Iteration #42 (55 evals): Loss: 0.003622 | RMSE Energy(low): 58.37 (57.96) meV/at | Forces(low): 75.32 (75.45) meV/A | Time/eval: 478.23 mcs/at
2022-06-06 19:46:47,159 - pyace.metrics_aggregator - INFO - Iteration #43 (56 evals): Loss: 0.003490 | RMSE Energy(low): 56.27 (56.15) meV/at | Forces(low): 74.14 (74.27) meV/A | Time/eval: 480.67 mcs/at
2022-06-06 19:46:48,455 - pyace.metrics_aggregator - INFO - Iteration #44 (57 evals): Loss: 0.003314 | RMSE Energy(low): 55.38 (55.84) meV/at | Forces(low): 72.68 (72.81) meV/A | Time/eval: 478.57 mcs/at
2022-06-06 19:46:49,861 - pyace.metrics_aggregator - INFO - Iteration #45 (58 evals): Loss: 0.003161 | RMSE Energy(low): 54.56 (55.36) meV/at | Forces(low): 71.69 (71.82) meV/A | Time/eval: 513.25 mcs/at
2022-06-06 19:46:51,155 - pyace.metrics_aggregator - INFO - Iteration #46 (59 evals): Loss: 0.003003 | RMSE Energy(low): 53.61 (54.06) meV/at | Forces(low): 70.20 (70.32) meV/A | Time/eval: 465.15 mcs/at
2022-06-06 19:46:52,455 - pyace.metrics_aggregator - INFO - Iteration #47 (60 evals): Loss: 0.002902 | RMSE Energy(low): 51.88 (52.19) meV/at | Forces(low): 68.03 (68.15) meV/A | Time/eval: 459.09 mcs/at
2022-06-06 19:46:53,758 - pyace.metrics_aggregator - INFO - Iteration #48 (61 evals): Loss: 0.002799 | RMSE Energy(low): 51.20 (51.47) meV/at | Forces(low): 66.89 (67.01) meV/A | Time/eval: 483.02 mcs/at
2022-06-06 19:46:55,053 - pyace.metrics_aggregator - INFO - Iteration #49 (62 evals): Loss: 0.002666 | RMSE Energy(low): 49.34 (49.97) meV/at | Forces(low): 66.55 (66.67) meV/A | Time/eval: 482.97 mcs/at
2022-06-06 19:46:56,457 - pyace.metrics_aggregator - INFO -
--------------------------------------------FIT STATS--------------------------------------------
Iteration: #50 Loss: Total: 2.4945e-03 (100%)
Energy: 6.4879e-04 ( 26%)
Force: 1.8456e-03 ( 74%)
L1: 9.2824e-08 ( 0%)
L2: 3.4948e-09 ( 0%)
Number of params./funcs: 1017/306 Avg. time: 471.34 mcs/at
-------------------------------------------------------------------------------------------------
Energy/at, meV/at Energy_low/at, meV/at Force, meV/A Force_low, meV/A
RMSE: 47.22 47.94 66.12 66.24
MAE: 18.77 19.26 36.60 36.73
MAX_AE: 380.38 380.38 962.40 962.40
-------------------------------------------------------------------------------------------------
2022-06-06 19:46:57,756 - pyace.metrics_aggregator - INFO - Iteration #51 (64 evals): Loss: 0.002374 | RMSE Energy(low): 45.58 (46.04) meV/at | Forces(low): 64.28 (64.40) meV/A | Time/eval: 484.40 mcs/at
2022-06-06 19:46:59,150 - pyace.metrics_aggregator - INFO - Iteration #52 (65 evals): Loss: 0.002190 | RMSE Energy(low): 42.85 (43.45) meV/at | Forces(low): 63.15 (63.26) meV/A | Time/eval: 497.06 mcs/at
2022-06-06 19:47:00,456 - pyace.metrics_aggregator - INFO - Iteration #53 (66 evals): Loss: 0.001980 | RMSE Energy(low): 39.80 (40.19) meV/at | Forces(low): 61.17 (61.27) meV/A | Time/eval: 483.69 mcs/at
2022-06-06 19:47:02,957 - pyace.metrics_aggregator - INFO - Iteration #54 (68 evals): Loss: 0.001879 | RMSE Energy(low): 38.08 (38.54) meV/at | Forces(low): 59.75 (59.86) meV/A | Time/eval: 535.32 mcs/at
2022-06-06 19:47:04,257 - pyace.metrics_aggregator - INFO - Iteration #55 (69 evals): Loss: 0.001799 | RMSE Energy(low): 35.67 (36.10) meV/at | Forces(low): 58.60 (58.70) meV/A | Time/eval: 480.75 mcs/at
2022-06-06 19:47:05,654 - pyace.metrics_aggregator - INFO - Iteration #56 (70 evals): Loss: 0.001718 | RMSE Energy(low): 33.70 (34.18) meV/at | Forces(low): 57.21 (57.31) meV/A | Time/eval: 508.70 mcs/at
2022-06-06 19:47:07,054 - pyace.metrics_aggregator - INFO - Iteration #57 (71 evals): Loss: 0.001671 | RMSE Energy(low): 33.32 (33.18) meV/at | Forces(low): 55.68 (55.78) meV/A | Time/eval: 525.67 mcs/at
2022-06-06 19:47:08,455 - pyace.metrics_aggregator - INFO - Iteration #58 (72 evals): Loss: 0.001607 | RMSE Energy(low): 33.03 (33.38) meV/at | Forces(low): 54.56 (54.66) meV/A | Time/eval: 525.81 mcs/at
2022-06-06 19:47:09,851 - pyace.metrics_aggregator - INFO - Iteration #59 (73 evals): Loss: 0.001525 | RMSE Energy(low): 32.67 (33.12) meV/at | Forces(low): 53.62 (53.72) meV/A | Time/eval: 520.25 mcs/at
2022-06-06 19:47:11,254 - pyace.metrics_aggregator - INFO - Iteration #60 (74 evals): Loss: 0.001437 | RMSE Energy(low): 31.52 (31.96) meV/at | Forces(low): 52.05 (52.14) meV/A | Time/eval: 526.30 mcs/at
2022-06-06 19:47:12,557 - pyace.metrics_aggregator - INFO - Iteration #61 (75 evals): Loss: 0.001358 | RMSE Energy(low): 29.44 (29.46) meV/at | Forces(low): 50.50 (50.59) meV/A | Time/eval: 482.77 mcs/at
2022-06-06 19:47:15,055 - pyace.metrics_aggregator - INFO - Iteration #62 (77 evals): Loss: 0.001315 | RMSE Energy(low): 29.03 (29.10) meV/at | Forces(low): 49.59 (49.68) meV/A | Time/eval: 489.01 mcs/at
2022-06-06 19:47:16,356 - pyace.metrics_aggregator - INFO - Iteration #63 (78 evals): Loss: 0.001262 | RMSE Energy(low): 28.16 (28.53) meV/at | Forces(low): 49.04 (49.13) meV/A | Time/eval: 478.26 mcs/at
2022-06-06 19:47:17,657 - pyace.metrics_aggregator - INFO - Iteration #64 (79 evals): Loss: 0.001220 | RMSE Energy(low): 27.38 (27.75) meV/at | Forces(low): 48.41 (48.50) meV/A | Time/eval: 476.58 mcs/at
2022-06-06 19:47:18,959 - pyace.metrics_aggregator - INFO - Iteration #65 (80 evals): Loss: 0.001176 | RMSE Energy(low): 26.21 (26.31) meV/at | Forces(low): 47.89 (47.98) meV/A | Time/eval: 453.29 mcs/at
2022-06-06 19:47:20,258 - pyace.metrics_aggregator - INFO - Iteration #66 (81 evals): Loss: 0.001124 | RMSE Energy(low): 24.88 (25.10) meV/at | Forces(low): 47.16 (47.25) meV/A | Time/eval: 457.33 mcs/at
2022-06-06 19:47:21,556 - pyace.metrics_aggregator - INFO - Iteration #67 (82 evals): Loss: 0.001075 | RMSE Energy(low): 23.00 (23.34) meV/at | Forces(low): 46.87 (46.96) meV/A | Time/eval: 478.28 mcs/at
2022-06-06 19:47:22,862 - pyace.metrics_aggregator - INFO - Iteration #68 (83 evals): Loss: 0.001039 | RMSE Energy(low): 22.20 (22.38) meV/at | Forces(low): 46.77 (46.85) meV/A | Time/eval: 475.96 mcs/at
2022-06-06 19:47:24,256 - pyace.metrics_aggregator - INFO - Iteration #69 (84 evals): Loss: 0.001008 | RMSE Energy(low): 20.56 (20.69) meV/at | Forces(low): 46.42 (46.50) meV/A | Time/eval: 490.79 mcs/at
2022-06-06 19:47:25,556 - pyace.metrics_aggregator - INFO - Iteration #70 (85 evals): Loss: 0.000974 | RMSE Energy(low): 19.72 (20.00) meV/at | Forces(low): 45.71 (45.79) meV/A | Time/eval: 456.03 mcs/at
2022-06-06 19:47:26,955 - pyace.metrics_aggregator - INFO - Iteration #71 (86 evals): Loss: 0.000935 | RMSE Energy(low): 19.62 (19.91) meV/at | Forces(low): 44.91 (44.99) meV/A | Time/eval: 507.05 mcs/at
2022-06-06 19:47:28,349 - pyace.metrics_aggregator - INFO - Iteration #72 (87 evals): Loss: 0.000894 | RMSE Energy(low): 17.89 (18.14) meV/at | Forces(low): 43.75 (43.83) meV/A | Time/eval: 521.90 mcs/at
2022-06-06 19:47:29,659 - pyace.metrics_aggregator - INFO - Iteration #73 (88 evals): Loss: 0.000869 | RMSE Energy(low): 16.89 (17.09) meV/at | Forces(low): 43.22 (43.30) meV/A | Time/eval: 480.55 mcs/at
2022-06-06 19:47:31,057 - pyace.metrics_aggregator - INFO - Iteration #74 (89 evals): Loss: 0.000842 | RMSE Energy(low): 15.78 (16.02) meV/at | Forces(low): 42.66 (42.74) meV/A | Time/eval: 510.29 mcs/at
2022-06-06 19:47:32,357 - pyace.metrics_aggregator - INFO - Iteration #75 (90 evals): Loss: 0.000818 | RMSE Energy(low): 14.28 (14.49) meV/at | Forces(low): 41.93 (42.01) meV/A | Time/eval: 482.64 mcs/at
2022-06-06 19:47:33,655 - pyace.metrics_aggregator - INFO - Iteration #76 (91 evals): Loss: 0.000785 | RMSE Energy(low): 13.08 (13.24) meV/at | Forces(low): 41.31 (41.38) meV/A | Time/eval: 453.83 mcs/at
2022-06-06 19:47:35,055 - pyace.metrics_aggregator - INFO - Iteration #77 (92 evals): Loss: 0.000765 | RMSE Energy(low): 11.29 (11.46) meV/at | Forces(low): 40.72 (40.79) meV/A | Time/eval: 507.79 mcs/at
2022-06-06 19:47:36,361 - pyace.metrics_aggregator - INFO - Iteration #78 (93 evals): Loss: 0.000742 | RMSE Energy(low): 11.43 (11.60) meV/at | Forces(low): 40.31 (40.38) meV/A | Time/eval: 482.20 mcs/at
2022-06-06 19:47:38,855 - pyace.metrics_aggregator - INFO - Iteration #79 (95 evals): Loss: 0.000730 | RMSE Energy(low): 10.76 (10.93) meV/at | Forces(low): 40.07 (40.15) meV/A | Time/eval: 489.60 mcs/at
2022-06-06 19:47:40,256 - pyace.metrics_aggregator - INFO - Iteration #80 (96 evals): Loss: 0.000714 | RMSE Energy(low): 10.41 (10.57) meV/at | Forces(low): 39.78 (39.85) meV/A | Time/eval: 497.52 mcs/at
2022-06-06 19:47:41,559 - pyace.metrics_aggregator - INFO - Iteration #81 (97 evals): Loss: 0.000691 | RMSE Energy(low): 9.20 (9.34) meV/at | Forces(low): 39.53 (39.60) meV/A | Time/eval: 466.70 mcs/at
2022-06-06 19:47:42,858 - pyace.metrics_aggregator - INFO - Iteration #82 (98 evals): Loss: 0.000676 | RMSE Energy(low): 8.23 (8.28) meV/at | Forces(low): 39.54 (39.61) meV/A | Time/eval: 458.44 mcs/at
2022-06-06 19:47:44,255 - pyace.metrics_aggregator - INFO - Iteration #83 (99 evals): Loss: 0.000660 | RMSE Energy(low): 7.80 (7.92) meV/at | Forces(low): 39.28 (39.35) meV/A | Time/eval: 492.99 mcs/at
2022-06-06 19:47:45,663 - pyace.metrics_aggregator - INFO - Iteration #84 (100 evals): Loss: 0.000646 | RMSE Energy(low): 7.99 (8.04) meV/at | Forces(low): 38.89 (38.96) meV/A | Time/eval: 522.75 mcs/at
2022-06-06 19:47:47,061 - pyace.metrics_aggregator - INFO - Iteration #85 (101 evals): Loss: 0.000630 | RMSE Energy(low): 7.99 (8.11) meV/at | Forces(low): 38.37 (38.44) meV/A | Time/eval: 501.36 mcs/at
2022-06-06 19:47:48,456 - pyace.metrics_aggregator - INFO - Iteration #86 (102 evals): Loss: 0.000617 | RMSE Energy(low): 7.75 (7.85) meV/at | Forces(low): 38.16 (38.23) meV/A | Time/eval: 495.81 mcs/at
2022-06-06 19:47:49,852 - pyace.metrics_aggregator - INFO - Iteration #87 (103 evals): Loss: 0.000606 | RMSE Energy(low): 7.29 (7.39) meV/at | Forces(low): 37.83 (37.90) meV/A | Time/eval: 511.02 mcs/at
2022-06-06 19:47:51,253 - pyace.metrics_aggregator - INFO - Iteration #88 (104 evals): Loss: 0.000596 | RMSE Energy(low): 6.93 (7.01) meV/at | Forces(low): 37.44 (37.51) meV/A | Time/eval: 529.66 mcs/at
2022-06-06 19:47:52,558 - pyace.metrics_aggregator - INFO - Iteration #89 (105 evals): Loss: 0.000592 | RMSE Energy(low): 6.29 (6.37) meV/at | Forces(low): 37.00 (37.06) meV/A | Time/eval: 487.41 mcs/at
2022-06-06 19:47:53,957 - pyace.metrics_aggregator - INFO - Iteration #90 (106 evals): Loss: 0.000586 | RMSE Energy(low): 5.97 (6.05) meV/at | Forces(low): 36.91 (36.98) meV/A | Time/eval: 498.27 mcs/at
2022-06-06 19:47:55,255 - pyace.metrics_aggregator - INFO - Iteration #91 (107 evals): Loss: 0.000577 | RMSE Energy(low): 5.68 (5.77) meV/at | Forces(low): 36.76 (36.82) meV/A | Time/eval: 483.75 mcs/at
2022-06-06 19:47:56,555 - pyace.metrics_aggregator - INFO - Iteration #92 (108 evals): Loss: 0.000571 | RMSE Energy(low): 5.55 (5.62) meV/at | Forces(low): 36.38 (36.45) meV/A | Time/eval: 484.83 mcs/at
2022-06-06 19:47:57,855 - pyace.metrics_aggregator - INFO - Iteration #93 (109 evals): Loss: 0.000565 | RMSE Energy(low): 5.29 (5.36) meV/at | Forces(low): 36.25 (36.32) meV/A | Time/eval: 483.54 mcs/at
2022-06-06 19:47:59,260 - pyace.metrics_aggregator - INFO - Iteration #94 (110 evals): Loss: 0.000555 | RMSE Energy(low): 5.08 (5.15) meV/at | Forces(low): 35.90 (35.96) meV/A | Time/eval: 501.67 mcs/at
2022-06-06 19:48:00,558 - pyace.metrics_aggregator - INFO - Iteration #95 (111 evals): Loss: 0.000543 | RMSE Energy(low): 4.64 (4.69) meV/at | Forces(low): 35.41 (35.47) meV/A | Time/eval: 484.67 mcs/at
2022-06-06 19:48:02,954 - pyace.metrics_aggregator - INFO - Iteration #96 (113 evals): Loss: 0.000538 | RMSE Energy(low): 4.67 (4.73) meV/at | Forces(low): 35.21 (35.27) meV/A | Time/eval: 533.54 mcs/at
2022-06-06 19:48:04,156 - pyace.metrics_aggregator - INFO - Iteration #97 (114 evals): Loss: 0.000531 | RMSE Energy(low): 4.69 (4.64) meV/at | Forces(low): 35.02 (35.08) meV/A | Time/eval: 440.57 mcs/at
2022-06-06 19:48:05,454 - pyace.metrics_aggregator - INFO - Iteration #98 (115 evals): Loss: 0.000522 | RMSE Energy(low): 4.62 (4.69) meV/at | Forces(low): 34.86 (34.92) meV/A | Time/eval: 455.92 mcs/at
2022-06-06 19:48:06,753 - pyace.metrics_aggregator - INFO - Iteration #99 (116 evals): Loss: 0.000513 | RMSE Energy(low): 4.84 (4.91) meV/at | Forces(low): 34.76 (34.83) meV/A | Time/eval: 483.89 mcs/at
2022-06-06 19:48:08,155 - pyace.metrics_aggregator - INFO -
--------------------------------------------FIT STATS--------------------------------------------
Iteration: #100 Loss: Total: 5.0805e-04 (100%)
Energy: 9.7166e-06 ( 2%)
Force: 4.9818e-04 ( 98%)
L1: 1.3966e-07 ( 0%)
L2: 9.4339e-09 ( 0%)
Number of params./funcs: 1017/306 Avg. time: 471.73 mcs/at
-------------------------------------------------------------------------------------------------
Energy/at, meV/at Energy_low/at, meV/at Force, meV/A Force_low, meV/A
RMSE: 5.28 5.35 34.67 34.73
MAE: 2.95 3.00 19.22 19.29
MAX_AE: 38.59 38.59 513.34 513.34
-------------------------------------------------------------------------------------------------
Warning: Maximum number of iterations has been exceeded.
Current function value: 0.000508
Iterations: 100
Function evaluations: 117
Gradient evaluations: 117
Fitting took 202.54 seconds
2022-06-06 19:48:08,501 - pyace.fitadapter - INFO - Optimization result(success=False, status=1, message=Maximum number of iterations has been exceeded., nfev=117, njev=117)
2022-06-06 19:48:08,502 - pyace.generalfit - INFO - Fitting cycle finished, final statistic:
2022-06-06 19:48:08,502 - pyace.metrics_aggregator - INFO -
--------------------------------------------Cycle last iteration:--------------------------------------------
Iteration: #101 Loss: Total: 5.0805e-04 (100%)
Energy: 9.7166e-06 ( 2%)
Force: 4.9818e-04 ( 98%)
L1: 1.3966e-07 ( 0%)
L2: 9.4339e-09 ( 0%)
Number of params./funcs: 1017/306 Avg. time: 471.73 mcs/at
-------------------------------------------------------------------------------------------------
Energy/at, meV/at Energy_low/at, meV/at Force, meV/A Force_low, meV/A
RMSE: 5.28 5.35 34.67 34.73
MAE: 2.95 3.00 19.22 19.29
MAX_AE: 38.59 38.59 513.34 513.34
-------------------------------------------------------------------------------------------------
2022-06-06 19:48:08,502 - pyace.generalfit - DEBUG - Update current_bbasisconfig.metadata = MapStringString{_fit_cycles: 1, _loss: 0.0005080467020294956, ace_evaluator_version: 2021.10.25, pacemaker_version: 0.2.7+49.gac4f57f, starttime: 2022-06-06 19:44:43.834661, tensorpot_version: 0+untagged.8.g783e6a6, user: jovyan}
2022-06-06 19:48:08,502 - pyace.generalfit - INFO - Select best fit #1 among all available (1)
2022-06-06 19:48:08,502 - pyace.generalfit - INFO - Best fitting attempt is #1
2022-06-06 19:48:08,535 - pyace.generalfit - INFO - Intermediate potential saved in interim_potential_best_cycle.yaml
2022-06-06 19:48:08,539 - pyace.generalfit - INFO - Fitting done
2022-06-06 19:48:08,540 - pyace.generalfit - DEBUG - Update metadata: MapStringString{_loss: 0.0005080467020294956, ace_evaluator_version: 2021.10.25, intermediate_time: 2022-06-06 19:48:08.503048, pacemaker_version: 0.2.7+49.gac4f57f, starttime: 2022-06-06 19:44:43.834661, tensorpot_version: 0+untagged.8.g783e6a6, user: jovyan}
2022-06-06 19:48:08,567 - pyace.generalfit - INFO - Final potential is saved to output_potential.yaml
2022-06-06 19:48:08,571 - root - INFO - Making predictions
2022-06-06 19:48:08,571 - root - INFO - For train data
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("concat_55:0", shape=(None,), dtype=int32), values=Tensor("concat_54:0", shape=(None, 3), dtype=float64), dense_shape=Tensor("gradient_tape/Cast_30:0", shape=(2,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
/srv/conda/envs/notebook/lib/python3.8/site-packages/tensorflow/python/framework/indexed_slices.py:448: UserWarning: Converting sparse IndexedSlices(IndexedSlices(indices=Tensor("gradient_tape/Reshape_937:0", shape=(None,), dtype=int32), values=Tensor("gradient_tape/Reshape_936:0", shape=(None, 3, 3), dtype=float64), dense_shape=Tensor("gradient_tape/Cast_65:0", shape=(3,), dtype=int32))) to a dense Tensor of unknown shape. This may consume a large amount of memory.
warnings.warn(
2022-06-06 19:48:25,052 - root - INFO - Computing nearest neighbours distances from 'tp_atoms'
2022-06-06 19:48:25,081 - root - INFO - Predictions are saved into train_pred.pckl.gzip (121.2KiB)
2022-06-06 19:48:25,081 - root - INFO - Ploting validation graphs
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:499: UserWarning: The value of the smallest subnormal for <class 'numpy.float64'> type is zero.
setattr(self, word, getattr(machar, word).flat[0])
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:89: UserWarning: The value of the smallest subnormal for <class 'numpy.float64'> type is zero.
return self._float_to_str(self.smallest_subnormal)
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:499: UserWarning: The value of the smallest subnormal for <class 'numpy.float32'> type is zero.
setattr(self, word, getattr(machar, word).flat[0])
/srv/conda/envs/notebook/lib/python3.8/site-packages/numpy/core/getlimits.py:89: UserWarning: The value of the smallest subnormal for <class 'numpy.float32'> type is zero.
return self._float_to_str(self.smallest_subnormal)
Loading B-basis from 'output_potential.yaml'
Converting to Ctilde-basis
Saving Ctilde-basis to 'output_potential.yace'
INFO:pyiron_log:run job: pacemaker_job id: 28, status: collect
INFO:pyiron_log:update master: None 28 modal
pr.job_table()
DEBUG:pyiron_log:sql_query: {'project': '/home/jovyan/potentials/potentials/03-ACE/fit_pace/%'}
id | status | chemicalformula | job | subjob | projectpath | project | timestart | timestop | totalcputime | computer | hamilton | hamversion | parentid | masterid | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 27 | created | None | small_AlLi_training_container | /small_AlLi_training_container | None | /home/jovyan/potentials/potentials/03-ACE/fit_pace/ | 2022-06-06 19:44:39.811609 | NaT | NaN | pyiron@jupyter-yury-2elysogorskiy#1 | TrainingContainer | 0.4 | None | None |
1 | 28 | finished | None | pacemaker_job | /pacemaker_job | None | /home/jovyan/potentials/potentials/03-ACE/fit_pace/ | 2022-06-06 19:44:41.494972 | 2022-06-06 19:48:30.460638 | 228.0 | pyiron@jupyter-yury-2elysogorskiy#1 | Pacemaker2022 | 0.2 | None | None |
Analyse fit#
plot loss function
plt.plot(job["output/log/loss"])
plt.xlabel("# iter")
plt.ylabel("Loss")
plt.loglog()
[]
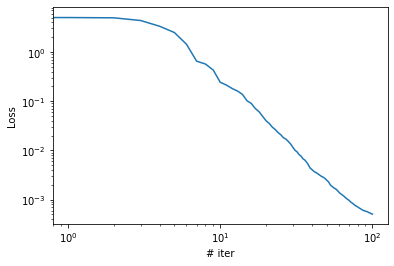
plot energy per atom RMSE
plt.plot(job["output/log/rmse_epa"])
plt.xlabel("# iter")
plt.ylabel("RMSE E, eV/atom")
plt.loglog()
[]
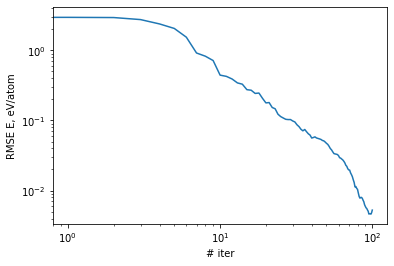
plot force component RMSE
plt.plot(job["output/log/rmse_f_comp"])
plt.xlabel("# iter")
plt.ylabel("RMSE F_i, eV/A")
plt.loglog()
[]
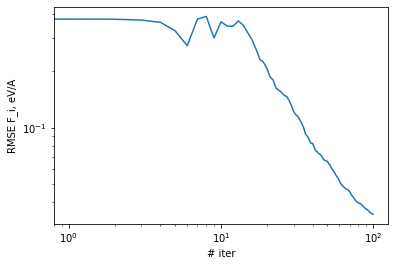
load DataFrame with predictions
ref_df = job.training_data
pred_df = job.predicted_data
plt.scatter(pred_df["energy_per_atom_true"],pred_df["energy_per_atom"])
plt.xlabel("DFT E, eV/atom")
plt.ylabel("ACE E, eV/atom")
Text(0, 0.5, 'ACE E, eV/atom')
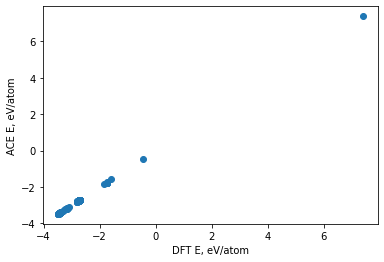
plt.scatter(ref_df["forces"],pred_df["forces"])
plt.xlabel("DFT F_i, eV/A")
plt.ylabel("ACE F_i, eV/A")
Text(0, 0.5, 'ACE F_i, eV/A')
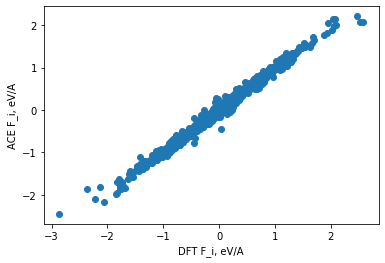
Check more in job.working_directory
/report folder
! ls {job.working_directory}/report
train_E-dE-dist.png train_EF-pairplots.png train_Fi-dFi-dist.png
train_E-dE-nn.png train_F-dF-dist.png
How actual potential file looks like ?#
output_potential.yaml
:
species:
# Pure Al interaction block
- speciesblock: Al
radbase: SBessel
radcoefficients: [[[1.995274603767268, -1.1940566258712266,...]]]
nbody:
# first order/ two-body functions = pair functions
- {type: Al Al, nr: [1], nl: [0], c: [2.0970219095074687, -3.9539202281610351]}
- {type: Al Al, nr: [2], nl: [0], c: [-1.8968648691718397, -2.3146574133175974]}
- {type: Al Al, nr: [3], nl: [0], c: [1.3504952496800906, 1.5291190439028692]}
- {type: Al Al, nr: [4], nl: [0], c: [0.040517989827027742, 0.11933504671036224]}
...
# second order/ three-body functions
- {type: Al Al Al, nr: [1, 1], nl: [0, 0], c: [0.57788490809100468, -1.8642896163994958]}
- {type: Al Al Al, nr: [1, 1], nl: [1, 1], c: [-1.0126646532267587, -1.2336078784112348]}
- {type: Al Al Al, nr: [1, 1], nl: [2, 2], c: [-0.19324470046809467, 0.63954472122968498]}
- {type: Al Al Al, nr: [1, 1], nl: [3, 3], c: [-0.22018334529075642, 0.32822679746839439]}
...
# fifth order/ six-body functions
- {type: Al Al Al Al Al Al, nr: [1, 1, 1, 1, 1], nl: [0, 0, 0, 0, 0], lint: [0, 0, 0], c: [-0.71...]}
# binary Al-Li interaction block
- speciesblock: Al Li
...
nbody:
- {type: Al Li, nr: [1], nl: [0], c: [0.91843424537280882, -2.4170371138562308]}
- {type: Al Li, nr: [2], nl: [0], c: [-0.88380210517336399, -0.97055273167339651]}
...
- {type: Al Al Al Li Li, nr: [1, 1, 1, 1], nl: [1, 1, 0, 0], lint: [0, 0], c: [-0.0050,...]}
...
# Pure Li interaction block
- speciesblock: Li
nbody:
...
- {type: Li Li Li, nr: [4, 4], nl: [3, 3], c: [-0.0059111333449957159, 0.035]}
- {type: Li Li Li Li, nr: [1, 1, 1], nl: [0, 0, 0], lint: [0], c: [0.210,...]}
...
# binary Al-Li interaction block
- speciesblock: Li Al
nbody:
...
- {type: Li Al Al, nr: [4, 4], nl: [3, 3], c: [0.014680736321211739, -0.030618474343919122]}
- {type: Li Al Li, nr: [1, 1], nl: [0, 0], c: [-0.22827705573988896, 0.28367909613209036]}
...
output_potential.yaml
is in B-basis form. For efficient LAMMPS implementaion it should be converted to so-called C-tilde
form. This is already done by pyiron
, but it could be also done manually by pace_yaml2yace
utility. Check here for more details
Get LAMMPS potential and try some calculations#
pace_lammps_potential = job.get_lammps_potential()
pace_lammps_potential
Config | Filename | Model | Name | Species | |
---|---|---|---|---|---|
0 | [pair_style pace\n, pair_coeff * * /home/jovyan/potentials/potentials/03-ACE/fit_pace/pacemaker_job_hdf5/pacemaker_job/output_potential.yace Al Li\n] | ACE | pacemaker_job | [Al, Li] |
lammps_job = pr.create.job.Lammps("pace_lammps", delete_existing_job=True)
lammps_job.structure=pr.create.structure.bulk("Al", cubic=True)
lammps_job.structure[0]='Li'
lammps_job.potential=pace_lammps_potential
murn=pr.create.job.Murnaghan("LiAl_murn")
murn.ref_job = lammps_job
murn.run()
murn.plot()
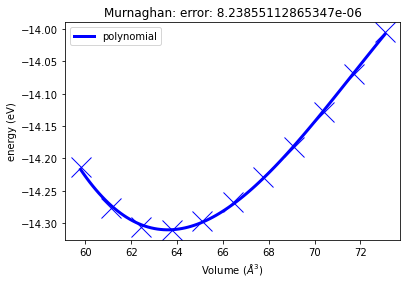
<AxesSubplot:title={'center':'Murnaghan: error: 8.23855112865347e-06'}, xlabel='Volume ($\\AA^3$)', ylabel='energy (eV)'>
Further reading#
Please read sources for more details: